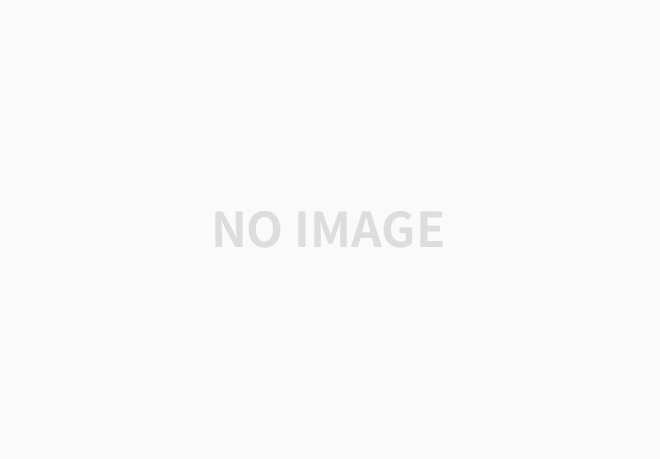
ํ์ด
๋ชจ๋ ๊ฒฝ์ฐ์ ์๋ฅผ DFS๋ก ํ์ํ๋ฉฐ ์ด์ ์ ๋ฐฉ๋ฌธํ ์ํ๋ฒณ์ธ ๊ฒฝ์ฐ ๊ฐ์ง์น๊ธฐ๋ฅผ ์งํํ์ฟ๋ค. ์ด ๋ ์ด์ ๋ฐฉ๋ฌธ ๋ ธ๋๋ฅผ ํ์ธํ๋ ๋ฐฉ๋ฒ์ผ๋ก ์ด๊ธฐ์๋ ArrayList๋ฅผ ์ฌ์ฉํ์ง๋ง ์๊ฐ์ด๊ณผ๊ฐ ๋ฐ์ํ์๊ณ ์ด์ ๋ํ ์ด์ ๊ฐ ArrayList์ remove ๊ณผ์ ์์ O(N)์ ์๊ฐ๋ณต์ก๋๊ฐ ๋ฐ์ํ๊ธฐ ๋๋ฌธ์ด๋ผ๊ณ ํ๋จํ์๋ค. ๊ทธ๋์ ์ด์ ๋ฐฉ๋ฌธ ์ํ๋ฒณ์ ์ ์งํ๋ ๋ฐฉ์์ StringBuilder๋ฅผ ์ฌ์ฉํ์๊ณ ๋ง์ง๋ง ์ธ๋ฑ์ค๋ฅผ ์ญ์ ํจ์ผ๋ก์จ ์๊ฐ ์ด๊ณผ๋ฅผ ํด๊ฒฐํ์๋ค.
ํ์ง๋ง ์ด ๋ฐฉ๋ฒ๋ ๋๋ฌด ๋๋ ค์ ์ด๋ฅผ ํ์ธํ๋ ๋ฐฉ๋ฒ์ผ๋ก ๋นํธ๋ง์คํน์ ์ฌ์ฉํ์๊ณ ๋ชจ๋ ๊ฒฝ์ฐ์ distance๋ฅผ ๋น๊ตํ๋ ๊ฒ์ด ์๋๋ผ ์ ๋งํ์ง ์์ ๊ฒฝ์ฐ์๋ง distance๋ฅผ ๋น๊ตํ๋๋ก ๊ฐ์ ํ์๋ค. ๋นํธ๋ง์คํน์ผ๋ก๋ ํ์ฌ ์ํ๋ฒณ - 'A'๋ฅผ ํด์ค์ผ๋ก์จ ํฌํจ ์ฌ๋ถ๋ฅผ ํ์ธํ์๋ค.
ArrayList
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
public class Solution1987 {
static BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
static int R, C, maxDistance = Integer.MIN_VALUE;
static String[][] map;
static boolean[][] check;
static ArrayList<String> previous;
static int[] dx = { 0, 1, 0, -1 };
static int[] dy = { 1, 0, -1, 0 };
public static void main(String[] args) throws IOException {
String[] input = br.readLine().split(" ");
R = Integer.parseInt(input[0]);
C = Integer.parseInt(input[1]);
map = new String[R][C];
check = new boolean[R][C];
for (int i = 0; i < R; i++) {
char[] line = br.readLine().toCharArray();
for (int j = 0; j < C; j++) {
map[i][j] = String.valueOf(line[j]);
}
}
previous = new ArrayList<String>();
traversal(0, 0, 1);
System.out.println(maxDistance);
}
private static void traversal(int x, int y, int distance) {
maxDistance = Math.max(maxDistance, distance);
check[x][y] = true;
previous.add(map[x][y]);
for (int i = 0; i < 4; i++) {
int nextX = x + dx[i], nextY = y + dy[i];
if (!isValid(nextX, nextY))
continue;
traversal(nextX, nextY, distance + 1);
}
previous.remove(map[x][y]);
check[x][y] = false;
}
static boolean isValid(int x, int y) {
if (x < 0 || y < 0 || x >= R || y >= C || previous.contains(map[x][y]) || check[x][y])
return false;
return true;
}
}
StringBuilder
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Main {
static BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
static int R, C, maxDistance = Integer.MIN_VALUE;
static String[][] map;
static boolean[][] check;
static StringBuilder previous;
static int[] dx = { 0, 1, 0, -1 };
static int[] dy = { 1, 0, -1, 0 };
public static void main(String[] args) throws IOException {
String[] input = br.readLine().split(" ");
R = Integer.parseInt(input[0]);
C = Integer.parseInt(input[1]);
map = new String[R][C];
check = new boolean[R][C];
for (int i = 0; i < R; i++) {
char[] line = br.readLine().toCharArray();
for (int j = 0; j < C; j++) {
map[i][j] = String.valueOf(line[j]);
}
}
previous = new StringBuilder(String.valueOf(map[0][0]));
traversal(0, 0, 1);
System.out.println(maxDistance);
}
private static void traversal(int x, int y, int distance) {
maxDistance = Math.max(maxDistance, distance);
for (int i = 0; i < 4; i++) {
int nextX = x + dx[i], nextY = y + dy[i];
if (!isValid(nextX, nextY))
continue;
check[nextX][nextY] = true;
previous.append(map[nextX][nextY]);
traversal(nextX, nextY, distance + 1);
check[nextX][nextY] = false;
previous = previous.deleteCharAt(previous.length()-1);
}
}
static boolean isValid(int x, int y) {
if (x < 0 || y < 0 || x >= R || y >= C || previous.toString().contains(map[x][y])
|| check[x][y])
return false;
return true;
}
}
Bitmasking
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Solution {
static BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
static int R, C, maxDistance = Integer.MIN_VALUE;
static char[][] map;
static int[] dx = { 0, 1, 0, -1 };
static int[] dy = { 1, 0, -1, 0 };
public static void main(String[] args) throws IOException {
String[] input = br.readLine().split(" ");
R = Integer.parseInt(input[0]);
C = Integer.parseInt(input[1]);
map = new char[R][C];
for (int i = 0; i < R; i++) {
char[] line = br.readLine().toCharArray();
for (int j = 0; j < C; j++) {
map[i][j] = line[j];
}
}
int start = map[0][0] - 'A';
traversal(0, 0, 1, 0|1<<start);
System.out.println(maxDistance);
}
private static void traversal(int x, int y, int distance, int flag) {
for (int i = 0; i < 4; i++) {
int nextX = x + dx[i], nextY = y + dy[i];
if (!isValid(nextX, nextY, flag)) {
maxDistance = Math.max(maxDistance, distance);
continue;
}
int bit = map[nextX][nextY] - 'A';
traversal(nextX, nextY, distance + 1, flag|1<<bit);
}
}
static boolean isValid(int x, int y, int flag) {
if (x < 0 || y < 0 || x >= R || y >= C )
return false;
int bit = map[x][y] - 'A';
if ((flag&1<<bit) != 0)
return false;
return true;
}
}
'๐ Algorithm > ๐ป ๋ฌธ์ ํ์ด' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[๋ฐฑ์ค] 1799. ๋น์ (0) | 2021.03.07 |
---|---|
[๋ฐฑ์ค] 1967. ํธ๋ฆฌ์ ์ง๋ฆ (0) | 2021.03.06 |
[๋ฐฑ์ค] 3109. ๋นต์ง (0) | 2021.02.19 |
[๋ฐฑ์ค] 15686. ์นํจ ๋ฐฐ๋ฌ (0) | 2021.02.19 |
[๋ฐฑ์ค] 9251. LCS (0) | 2021.02.10 |
๋๊ธ